In-memory caching stands as a cornerstone technique in optimizing the performance and scalability of ASP.NET Core applications. By storing frequently accessed data in memory, developers can drastically reduce the need to fetch information from slower data sources such as databases or external APIs. This leads to faster response times, improved resource utilization, and ultimately, a superior user experience. In this comprehensive guide, we’ll delve deep into the intricacies of in-memory caching, exploring its benefits, setup process, implementation strategies, and best practices.
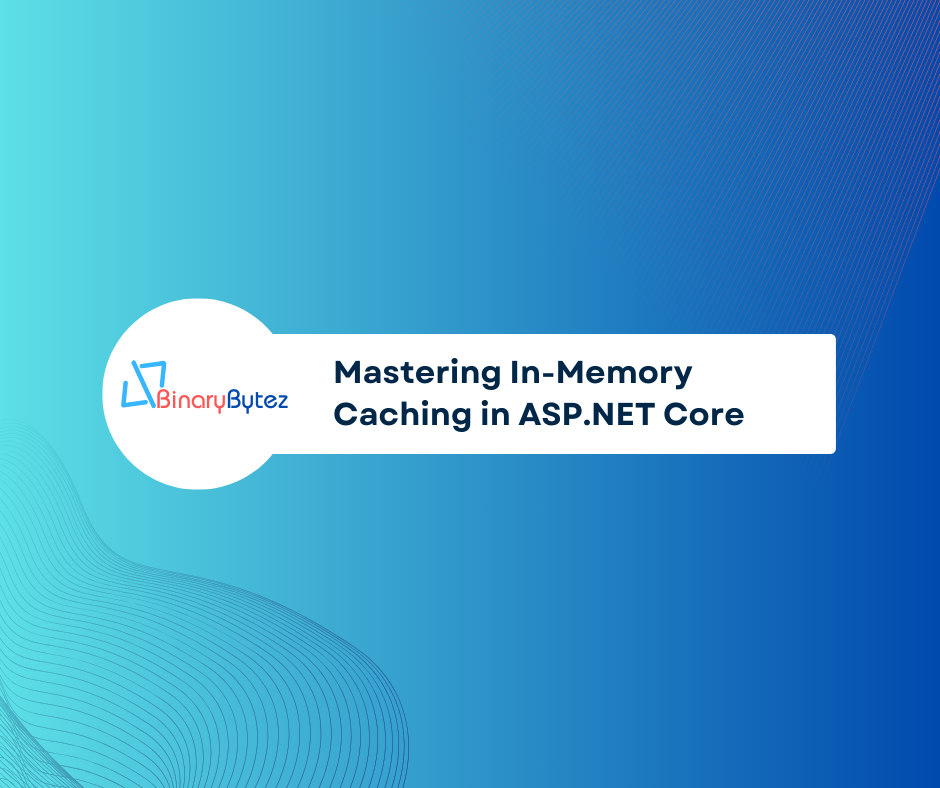
Understanding the Significance of In-Memory Caching:
Accelerated Performance
The primary advantage of in-memory caching lies in its ability to boost application performance. Retrieving data from memory is inherently faster than fetching it from disk or over the network. Consequently, in-memory caching significantly reduces latency, ensuring that users receive timely responses to their requests.
Reduced Load on Data Sources
By caching frequently accessed data, ASP.NET Core applications can alleviate the burden on external data sources, such as databases or web services. This reduction in load translates to improved scalability, as the application can handle more concurrent users without compromising performance.
Enhanced User Experience
In-memory caching contributes to an enhanced user experience by providing quick access to frequently requested information. Whether it’s product listings, user profiles, or dynamic content, caching ensures that data is readily available, resulting in a smoother and more responsive application interface.
When to Use In-Memory Caching:
While in-memory caching offers numerous benefits, it’s essential to use it judiciously and considerately. Here are some scenarios and considerations for leveraging in-memory caching effectively in ASP.NET Core applications:
Frequently Accessed Data
In-memory caching is most beneficial for data that is accessed frequently but changes infrequently. Examples include reference data, configuration settings, and static content. Caching such data in memory reduces the overhead of repeated database or file system accesses, leading to significant performance improvements.
High-Volume Read Operations
Applications with high-volume read operations, such as e-commerce platforms with product listings or content management systems with articles, can benefit greatly from in-memory caching. By caching frequently accessed data, these applications can handle large numbers of concurrent users while maintaining responsiveness and scalability.
Expensive Computations or Queries
In-memory caching can also be valuable for caching the results of expensive computations or database queries. By caching the computed or queried results, subsequent requests for the same data can be served quickly from memory, avoiding the need to repeat the costly operation.
User Session Data
For applications that store user-specific data during a session, such as shopping cart contents or user preferences, in-memory caching can provide a fast and efficient mechanism for managing session state. By caching session data in memory, the application can minimize latency and improve user experience.
Temporary Data Storage
In-memory caching can serve as a temporary data storage solution for transient data that doesn’t need to be persisted long-term. Examples include temporary authentication tokens, short-lived cache keys, or data used for the duration of a user session. Caching such data in memory can help reduce database load and improve application performance.
Setting Up In-Memory Caching in ASP.NET Core:
Configuring Services
Enabling in-memory caching in an ASP.NET Core application begins with configuring the necessary services. This can be achieved in the Startup.cs
file within the ConfigureServices
method.
public void ConfigureServices(IServiceCollection services)
{
services.AddMemoryCache(); // Add caching services
// Additional service configurations...
}
By invoking the AddMemoryCache()
method, developers incorporate the essential caching services into the application’s service container.
Injecting IMemoryCache
To utilize in-memory caching within various components of the application, developers need to inject the IMemoryCache
interface. This is typically done through constructor injection in controllers, services, or other relevant classes.
using Microsoft.Extensions.Caching.Memory;
public class ProductController : ControllerBase
{
private readonly IMemoryCache _cache;
public ProductController(IMemoryCache cache)
{
_cache = cache;
}
// Controller actions...
}
Implementing In-Memory Caching Strategies:
With in-memory caching configured and IMemoryCache
injected into the application components, developers can proceed to implement caching logic tailored to their specific use cases. Let’s explore a detailed example of caching product data in an ASP.NET Core web API.
Example: Caching Product Data
Suppose we have an endpoint in our web API for retrieving product details by ID. We want to cache the product data to improve performance and reduce database load.
[HttpGet("{id}")]
public async Task<IActionResult> GetProduct(int id)
{
// Attempt to retrieve the product from the cache
if (_cache.TryGetValue($"Product_{id}", out Product cachedProduct))
{
return Ok(cachedProduct); // Return the cached product
}
// If not found in cache, fetch the product from the data source (e.g., database or external API)
Product product = await _productService.GetProductById(id);
if (product != null)
{
// Cache the product with an expiration time of 5 minutes
_cache.Set($"Product_{id}", product, TimeSpan.FromMinutes(5));
return Ok(product); // Return the fetched product
}
else
{
return NotFound(); // Product not found
}
}
In this example:
- We attempt to retrieve the product with the given ID from the cache using
TryGetValue()
. - If the product is found in the cache, it’s returned directly from memory.
- If not found, we fetch the product from the data source (e.g., database or external API) and cache it using
Set()
with an expiration time of 5 minutes.
Syntax Examples:
Here are examples of syntax for adding, removing, and other operations related to in-memory caching in ASP.NET Core using the IMemoryCache
interface:
Adding Data to Cache:
_cache.Set("CacheKey", cachedData, TimeSpan.FromMinutes(10)); // Cache data with a key and expiration time
Retrieving Data from Cache:
if (_cache.TryGetValue("CacheKey", out CachedDataType cachedData))
{
// Data found in cache, use cachedData
}
else
{
// Data not found in cache, fetch from source and cache it
}
Removing Data from Cache:
_cache.Remove("CacheKey"); // Remove data from cache by key
Clearing All Cached Data:
_cache.Clear(); // Clear all data from cache
Checking if Data Exists in Cache:
if (_cache.TryGetValue("CacheKey", out CachedDataType cachedData))
{
// Data exists in cache
}
else
{
// Data does not exist in cache
}
These syntax examples demonstrate how to perform common operations related to in-memory caching in ASP.NET Core using the IMemoryCache
interface. From adding and retrieving data to removing entries and configuring cache options, developers can effectively utilize in-memory caching to optimize performance and enhance the user experience of their applications.
Conclusion:
In-memory caching represents a cornerstone technique for optimizing the performance and scalability of ASP.NET Core applications. By strategically caching frequently accessed data in memory, developers can minimize latency, reduce load on data sources, and deliver a superior user experience. Armed with a solid understanding of in-memory caching concepts and implementation strategies, developers can leverage this powerful tool to build high-performing and responsive applications that meet the demands of modern users.