Introduction:
Performance optimization and monitoring play a vital role in ensuring the efficiency, scalability, and reliability of ASP.NET Core applications. In this comprehensive guide, we’ll delve into various techniques, best practices, and tools for optimizing performance and monitoring the health of ASP.NET Core applications, accompanied by detailed examples for each concept.
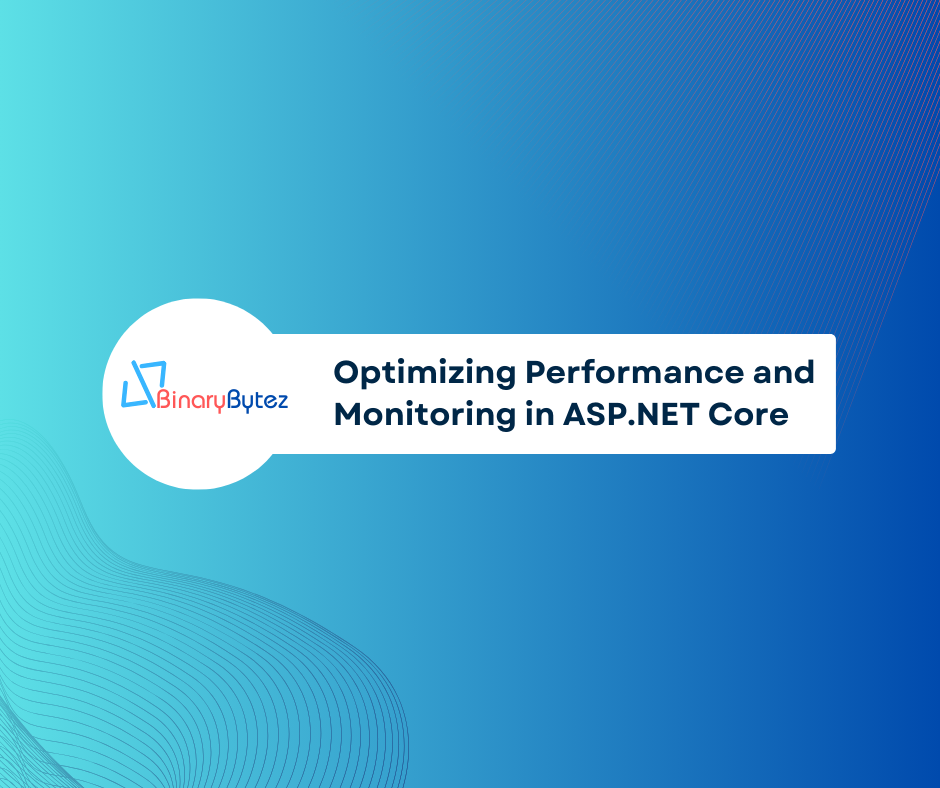
Optimizing Performance:
1. Efficient Database Querying with Entity Framework Core:
Efficient database querying is crucial for improving application performance. With Entity Framework Core, we can optimize database access by minimizing the number of round-trips and optimizing query execution. Example:
// Before Optimization
var products = dbContext.Products.Where(p => p.CategoryId == categoryId).ToList();
// After Optimization
var products = dbContext.Products.AsNoTracking().Where(p => p.CategoryId == categoryId).ToList();
In the optimized query, we use AsNoTracking()
to disable change tracking for read-only operations, resulting in improved performance.
2. Caching Strategies for Improved Performance:
Caching frequently accessed data can significantly reduce database round-trips and improve application response times. ASP.NET Core provides built-in caching mechanisms that we can leverage to implement caching strategies effectively. Example:
public async Task<List<Product>> GetProductsAsync(int categoryId)
{
var cacheKey = $"products_{categoryId}";
if (!_cache.TryGetValue(cacheKey, out List<Product> products))
{
products = await dbContext.Products.Where(p => p.CategoryId == categoryId).ToListAsync();
_cache.Set(cacheKey, products, TimeSpan.FromMinutes(10)); // Cache for 10 minutes
}
return products;
}
In this example, we cache the products retrieved based on the category ID for a specified duration, enhancing application performance.
3. Asynchronous Programming for Scalability:
Asynchronous programming enables applications to handle multiple concurrent operations efficiently, leading to improved scalability and responsiveness. By utilizing async/await keywords in ASP.NET Core, we can execute asynchronous operations seamlessly. Example:
public async Task<ActionResult> GetProductsAsync()
{
var products = await dbContext.Products.ToListAsync();
return Ok(products);
}
This example demonstrates retrieving products asynchronously from the database, enhancing the scalability of the application.
4. Compression for Network Efficiency:
Compressing HTTP responses can significantly reduce bandwidth usage and improve network efficiency. ASP.NET Core supports response compression middleware, allowing us to compress HTTP responses before sending them to clients. Example:
public void Configure(IApplicationBuilder app)
{
app.UseResponseCompression();
}
By enabling response compression middleware, we can reduce data transfer sizes and improve overall network efficiency.
5. Lazy Loading for Improved Page Load Times:
Lazy loading is a technique used to defer loading resources until they are needed, enhancing initial page load times and improving user experience. In ASP.NET Core applications, we can implement lazy loading for resources such as images and scripts. Example:
<img src="placeholder.jpg" data-src="image.jpg" loading="lazy" alt="Lazy Loaded Image">
This example illustrates lazy loading of an image by specifying the actual image source in the data-src
attribute, reducing initial page load times.
Monitoring and Diagnostics:
1. Application Insights for Real-time Monitoring:
Application Insights is a powerful tool provided by Azure for monitoring and diagnosing ASP.NET Core applications in real time. By integrating Application Insights into our application, we can gain valuable insights into application performance, availability, and usage. Example:
public void ConfigureServices(IServiceCollection services)
{
services.AddApplicationInsightsTelemetry(Configuration["InstrumentationKey"]);
}
By adding Application Insights telemetry, we can monitor various metrics such as request rates, response times, and server resource utilization, enabling proactive performance monitoring and diagnostics.
2. Logging and Error Handling with Serilog:
Logging is essential for capturing and analyzing application events, errors, and diagnostic information. Serilog is a popular logging library for ASP.NET Core applications, offering flexible configuration and various output sinks for logging data. Example:
public void ConfigureLogger()
{
Log.Logger = new LoggerConfiguration()
.WriteTo.File("log.txt")
.CreateLogger();
}
By configuring Serilog to log to a file, we can capture application events and errors for troubleshooting and analysis, enhancing the overall monitoring and diagnostics capabilities of the application.
3. Health Checks for Application Health Monitoring:
Health checks are endpoints that provide information about the health of an application and its dependencies. By implementing health checks in ASP.NET Core applications, we can monitor the health and availability of critical components, such as databases, external services, and internal dependencies. Example:
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks();
}
This example demonstrates adding health checks to the ASP.NET Core application, enabling continuous monitoring of the application’s health and readiness.
4. Profiling and Performance Analysis with MiniProfiler:
MiniProfiler is a lightweight profiling tool that helps identify performance bottlenecks in ASP.NET Core applications. By integrating MiniProfiler into our application, we can profile individual requests and identify areas for performance optimization. Example:
public async Task<ActionResult> GetProductsAsync()
{
using (MiniProfiler.Current.Step("GetProductsAsync"))
{
var products = await dbContext.Products.ToListAsync();
return Ok(products);
}
}
By wrapping the database query in a MiniProfiler step, we can measure the execution time and identify potential performance bottlenecks, enhancing the overall performance tuning and monitoring capabilities of the application.
Conclusion:
Optimizing performance and monitoring the health of ASP.NET Core applications are essential for delivering a seamless user experience and ensuring the reliability and scalability of the application. By implementing the techniques and best practices outlined in this guide, ASP.NET Core developers can optimize application performance, enhance scalability, and proactively monitor and diagnose issues, ensuring the smooth operation of their applications in production environments.