In the competitive landscape of software development, performance is a crucial factor that can make or break the success of your .NET applications. Whether you’re building enterprise-level web applications, APIs, or microservices, optimizing performance is essential for delivering a seamless user experience and maintaining competitiveness in the market. In this comprehensive guide, we’ll explore 15 best practices that can help you maximize the performance of your .NET applications, complete with detailed explanations and code examples.
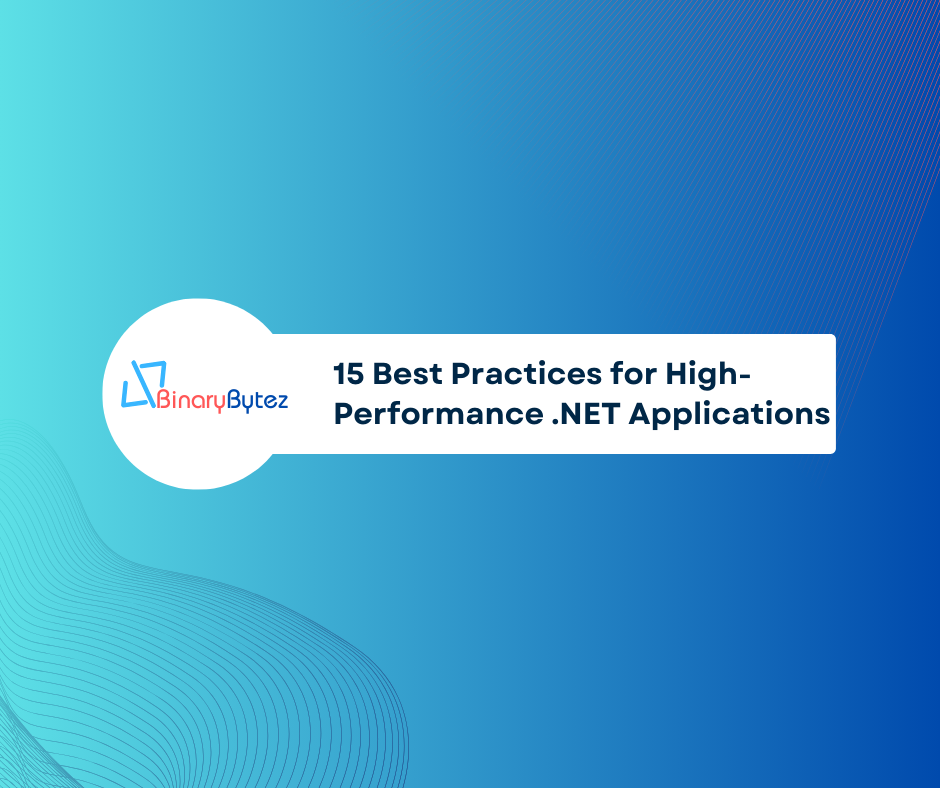
1. Utilize Caching
Caching is a fundamental technique for improving the performance of .NET applications by storing frequently accessed data in memory. This reduces the need to retrieve data from slower data sources such as databases. In .NET, you can leverage the MemoryCache
class from the Microsoft.Extensions.Caching.Memory
namespace.
public class ProductController : ControllerBase
{
private readonly IMemoryCache _cache;
private readonly IProductRepository _productRepository;
public ProductController(IMemoryCache cache, IProductRepository productRepository)
{
_cache = cache;
_productRepository = productRepository;
}
[HttpGet("{id}")]
public IActionResult GetProduct(int id)
{
var cacheKey = $"Product_{id}";
if (!_cache.TryGetValue(cacheKey, out Product product))
{
product = _productRepository.GetProduct(id);
if (product != null)
{
// Cache for 10 minutes
_cache.Set(cacheKey, product, TimeSpan.FromMinutes(10));
}
}
return Ok(product);
}
}
In this example, the MemoryCache
is used to store product data retrieved from the database. If the product is not found in the cache, it’s fetched from the database and then stored in the cache with an expiration time of 10 minutes.
2. Optimize Hot Code Paths
Identify and optimize hot code paths, which are sections of code that are frequently executed and contribute significantly to the overall runtime of your application. By optimizing these paths, you can achieve noticeable performance improvements.
public class OrderProcessor
{
public void ProcessOrder(Order order)
{
foreach (var item in order.Items)
{
// Optimize this section for performance
}
}
}
In this example, the ProcessOrder
method represents a hot code path responsible for processing order details. It should be optimized for performance, possibly by using more efficient algorithms or data structures within the loop.
3. Use Asynchronous APIs
Leverage asynchronous programming to handle multiple operations concurrently, improving scalability and responsiveness. Asynchronous APIs in .NET can be utilized using the async
and await
keywords.
public async Task<IActionResult> GetDataAsync()
{
var data = await _dataService.GetDataAsync();
return Ok(data);
}
In this example, the GetDataAsync
method asynchronously retrieves data from a service. This allows the application to continue executing other tasks while waiting for the data to be fetched.
4. Asynchronize Hot Code Paths
Convert hot code paths to asynchronous operations to further enhance concurrency and responsiveness, especially in performance-critical sections of your code.
public async Task ProcessOrdersAsync(List<Order> orders)
{
foreach (var order in orders)
{
// Asynchronously process each order
await ProcessOrderAsync(order);
}
}
private async Task ProcessOrderAsync(Order order)
{
// Asynchronously process order details
await Task.Delay(100); // Example asynchronous operation
}
In performance-critical code paths, consider making asynchronous versions of methods to allow for non-blocking execution and improved concurrency.
5. Implement Pagination for Large Collections
When dealing with large datasets, implement pagination to fetch data in smaller chunks, reducing memory consumption and improving performance.
public IActionResult GetProducts(int page = 1, int pageSize = 10)
{
var products = _productRepository.GetProducts()
.Skip((page - 1) * pageSize)
.Take(pageSize)
.ToList();
return Ok(products);
}
In this example, the GetProducts
method retrieves a paginated list of products from the repository, allowing the client to request data in smaller chunks.
6. Prefer IAsyncEnumerable
Use IAsyncEnumerable<T>
for asynchronous enumeration of collections to prevent synchronous blocking and improve efficiency, especially with large datasets.
public async IAsyncEnumerable<int> GetNumbersAsync()
{
for (int i = 0; i < 10; i++)
{
await Task.Delay(100); // Simulate asynchronous operation
yield return i;
}
}
7. Cache Large Objects and Use ArrayPool
Optimize memory usage by caching frequently used large objects and managing large arrays with ArrayPool<T>
.
public void ProcessLargeArray()
{
var largeArray = ArrayPool<int>.Shared.Rent(100000);
// Process large array
ArrayPool<int>.Shared.Return(largeArray);
}
8. Optimize Data Access and I/O
Minimize roundtrips and latency by optimizing data access and I/O operations, such as database queries and external API calls.
public async Task<IActionResult> GetCachedDataAsync()
{
var cachedData = await _cache.GetOrCreateAsync("cached_data_key", async entry =>
{
// Retrieve data from database or external service
var data = await _dataService.GetDataAsync();
// Cache for 15 minutes
entry.AbsoluteExpirationRelativeToNow = TimeSpan.FromMinutes(15);
return data;
});
return Ok(cachedData);
}
9. Use HttpClientFactory
Manage and pool HTTP connections efficiently with HttpClientFactory
to improve performance when making HTTP requests.
public async Task<IActionResult> GetRemoteDataAsync()
{
var client = _httpClientFactory.CreateClient();
var response = await client.GetAsync("https://api.example.com/data");
if (response.IsSuccessStatusCode)
{
var data = await response.Content.ReadAsStringAsync();
return Ok(data);
}
else
{
return StatusCode((int)response.StatusCode);
}
}
10. Profile and Optimize Middleware Components
Profile and optimize frequently-called middleware components to minimize their impact on request processing time and overall application performance.
// Example middleware component
public class LoggingMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<LoggingMiddleware> _logger;
public LoggingMiddleware(RequestDelegate next, ILogger<LoggingMiddleware> logger)
{
_next = next;
_logger = logger;
}
public async Task Invoke(HttpContext context)
{
// Log request information
_logger.LogInformation($"Request: {context.Request.Path}");
await _next(context);
}
}
11. Use Background Services for Long-Running Tasks
Offload long-running tasks to background services to maintain application responsiveness and prevent blocking of the main application thread.
public class EmailSenderService : BackgroundService
{
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
// Check for pending emails and send them
await SendPendingEmailsAsync();
// Wait for some time before checking again
await Task.Delay(TimeSpan.FromMinutes(5), stoppingToken);
}
}
}
12. Compress Responses to Reduce Payload Sizes
Enable response compression to reduce the size of HTTP responses, minimizing network bandwidth usage and improving overall application performance, especially for web applications.
// Configure response compression in Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression(options =>
{
options.EnableForHttps = true;
options.MimeTypes = new[] { "text/plain", "text/html", "application/json" };
});
}
13. Stay Updated with Latest ASP.NET Core Releases
Ensure your application is up-to-date with the latest ASP.NET
Core releases to leverage performance improvements and new features provided by the framework.
14. Avoid Concurrent Access to HttpContext
Avoid concurrent access to HttpContext
as it is not thread-safe. Access to HttpContext
should be synchronized to prevent race conditions and ensure application stability.
// Example usage in controller action
public IActionResult GetUserData()
{
var userId = HttpContext.User.FindFirst(ClaimTypes.NameIdentifier)?.Value;
// Retrieve user data using userId
return Ok(userData);
}
15. Handle HttpRequest.ContentLength Appropriately
Handle scenarios where HttpRequest.ContentLength
is null to ensure robustness and reliability in request processing, especially when dealing with incoming HTTP requests.
// Example usage in controller action
public async Task<IActionResult> ProcessFormDataAsync()
{
if (Request.ContentLength == null)
{
return BadRequest("Content length is not provided");
}
// Process form data
return Ok();
}
By implementing these 15 best practices in your .NET applications, you can significantly enhance their performance, scalability, and user experience, enabling you to meet the demands of modern, high-performance software development. Remember, performance optimization is an ongoing process, so continuously monitor and refine your application to ensure it remains optimized for maximum efficiency.