Understanding .NET Object Mapping: In the world of .NET development, working with objects and data transformation is a common task. To simplify this process, various object mapping libraries have emerged. In this guide, I’ll explore four popular .NET object mapping libraries: AutoMapper, TinyMapper, Mapster, and Inline Mapping. I’ll also provide examples and delve deeper into scenarios where each library shines.
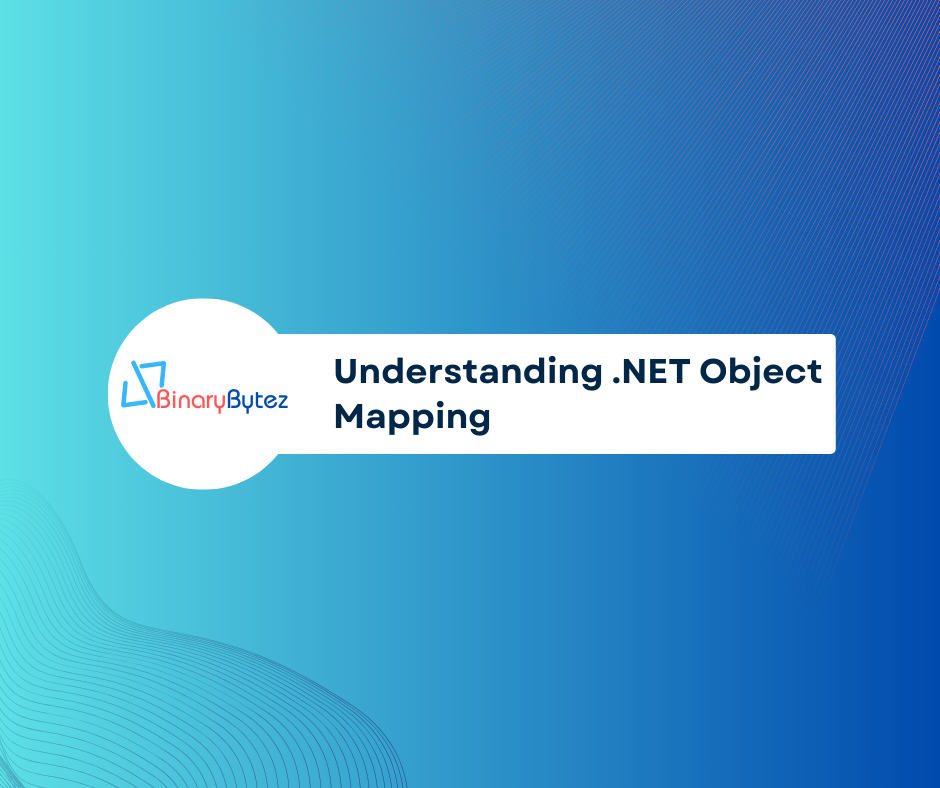
Introduction to Object Mapping
Object mapping, also known as object-to-object mapping, is the process of converting data from one object type to another. This is particularly useful when you need to transform data between different layers of your application or when integrating with external services or databases.
Common Use Cases
a. Database Mapping
When working with databases, you often need to map the result of a database query to a .NET object or entity. Similarly, you might need to map .NET objects back to the database structure for data storage.
b. API Integration
When communicating with external APIs, you may receive data in JSON or XML format that needs to be mapped to .NET objects. Conversely, you might need to serialize .NET objects into the required format for API requests.
c. ViewModel Creation
In web applications, it’s common to map domain models to view models for rendering in views. This mapping ensures that sensitive or unnecessary data is not exposed to the client.
Popular .NET Mappers
1. AutoMapper
When to Use AutoMapper
AutoMapper is a widely adopted and feature-rich object mapping library for .NET. It simplifies complex mappings and provides a fluent configuration API. Here are some scenarios where AutoMapper is an excellent choice:
- Complex Mappings: Use AutoMapper when dealing with complex mappings between objects with different structures.
- Configuration Flexibility: It offers extensive configuration options for fine-grained control over mappings.
- Large-Scale Projects: In large projects with many mappings, AutoMapper helps maintain a clear and organized mapping setup.
Example
// Configuration
var config = new MapperConfiguration(cfg => {
cfg.CreateMap<SourceClass, DestinationClass>();
});
// Mapping
var mapper = config.CreateMapper();
var destination = mapper.Map<SourceClass, DestinationClass>(source);
2. TinyMapper
When to Use TinyMapper
TinyMapper is a lightweight and high-performance object mapping library for .NET. It focuses on simplicity and speed. Consider using TinyMapper in the following situations:
- Performance-Critical Applications: When performance is crucial, TinyMapper’s lightweight nature shines.
- Simple Mappings: For straightforward one-to-one property mappings without complex configurations.
- Quick Setup: TinyMapper is easy to set up and use, making it suitable for small to medium projects.
Example
// Mapping
TinyMapper.Bind<SourceClass, DestinationClass>();
var destination = TinyMapper.Map<DestinationClass>(source);
3. Mapster
When to Use Mapster
Mapster is another lightweight and easy-to-use object mapping library for .NET. It emphasizes simplicity and performance. Here are scenarios where Mapster is a good fit:
- Simple to Moderate Mappings: When you need a balance between simplicity and flexibility.
- Performance-Oriented Applications: Mapster’s performance is suitable for applications with high data transformation requirements.
- Minimal Setup: Mapster requires minimal setup and configuration.
Example
// Mapping
var destination = source.Adapt<DestinationClass>();
4. Inline Mapping (Manual Mapping)
When to Use Inline Mapping
Inline mapping, also known as manual mapping, involves manually writing code to perform the mapping. While it doesn’t rely on an external library, it requires more manual effort. Use inline mapping in these scenarios:
- Full Control: When you want complete control over the mapping process and need to handle custom or complex transformations.
- Simple Mappings: For cases where using a library might be overkill and the mapping is straightforward.
- Small-Scale Projects: In smaller projects where the overhead of a mapping library isn’t justified.
Example
// Inline Mapping
var destination = new DestinationClass
{
Property1 = source.Property1,
Property2 = source.Property2
// ...
};
Comparison and Choosing the Right Mapper
Choosing the right object mapping library for your .NET project depends on your specific requirements. Here’s a summarized comparison:
- AutoMapper: Ideal for complex mappings and scenarios where you need a high degree of configuration control. It’s feature-rich but may be overkill for simple mappings.
- TinyMapper: Best for scenarios where performance is crucial due to its lightweight nature. It’s simpler to set up and use but has fewer features than AutoMapper.
- Mapster: Similar to TinyMapper, it’s lightweight and easy to use. Choose Mapster for straightforward mappings and when performance is essential.
- Inline Mapping: Suitable for simple mappings or when you want full control over the mapping process. It’s the most manual option but offers complete flexibility.
Conclusion
Choosing the right object mapping library for your .NET project depends on your specific needs. Consider factors like complexity, performance, and your familiarity with the library. Whether you opt for AutoMapper, TinyMapper, Mapster, or inline mapping, these tools will help streamline your data transformation tasks, making your code more maintainable and efficient.