Introduction
LINQ (Language-Integrated Query) and Lambda expressions are powerful features in C# that allow developers to query and manipulate data in a concise and expressive manner. While both are used for similar purposes, they have distinct syntaxes and use cases. This document aims to provide a comprehensive comparison between LINQ and Lambda expressions in C#, along with code examples to illustrate their usage.
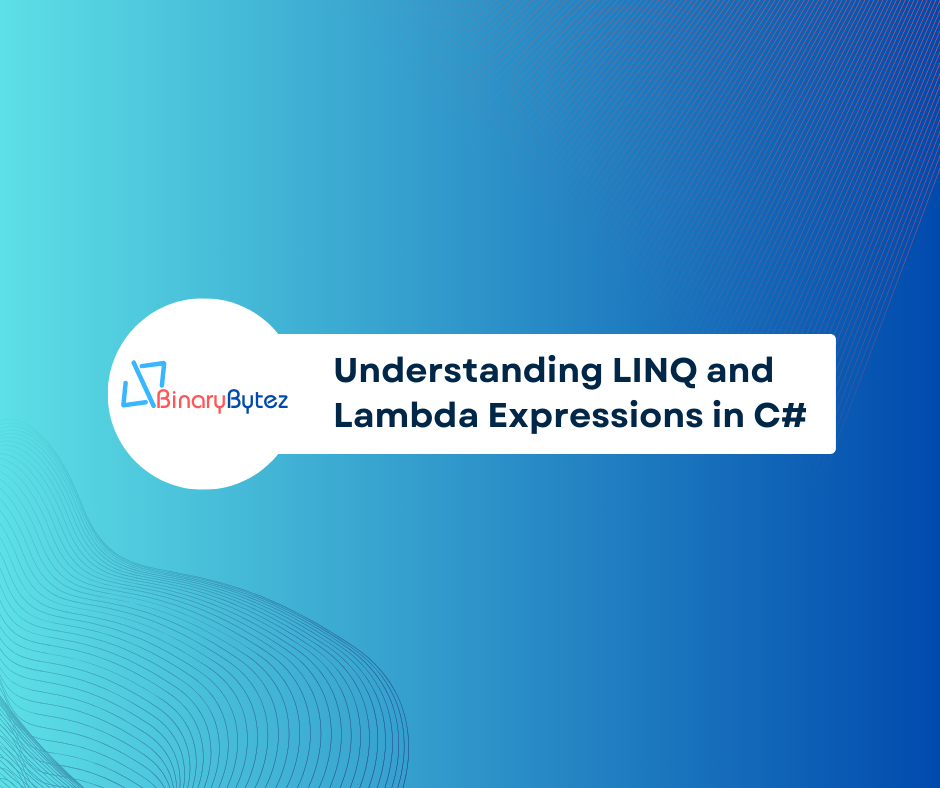
LINQ (Language-Integrated Query):
- Definition: LINQ is a set of features in C# that enables developers to query data from different data sources using a SQL-like syntax directly within the C# language.
- Syntax: LINQ syntax consists of keywords such as
from
,where
,select
,group by
,order by
, etc., which resemble SQL syntax. - Use Cases: LINQ is commonly used for querying data from collections (such as arrays, lists, and dictionaries), databases (using Entity Framework or LINQ to SQL), XML files, and other data sources.
- Code Example: Querying a list of objects using LINQ.
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = from num in numbers
where num % 2 == 0
select num;
Lambda Expressions:
- Definition: Lambda expressions are anonymous functions that allow developers to write inline delegate functions without explicitly defining a method.
- Syntax: Lambda expressions consist of the
=>
(arrow) operator, parameters, and an expression or statement block. - Use Cases: Lambda expressions are commonly used for sorting, filtering, and transforming data within LINQ queries, as well as in LINQ extension methods and delegates.
- Code Example: Filtering a list of objects using Lambda expressions.
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = numbers.Where(num => num % 2 == 0);
Key Differences:
- Syntax: LINQ uses a declarative query syntax resembling SQL, while Lambda expressions use a more concise and functional syntax.
- Readability: LINQ queries are often more readable and intuitive for complex queries involving multiple operations, while Lambda expressions are preferred for simple and single-purpose operations.
- Flexibility: Lambda expressions provide more flexibility and can be used in various contexts beyond LINQ queries, such as delegates, event handlers, and LINQ extension methods.
Let’s expand on the previous examples and provide multiple scenarios to illustrate the usage of LINQ and Lambda expressions in C#.
1. Filtering a List of Objects:
Using LINQ:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = from num in numbers
where num % 2 == 0
select num;
Using Lambda Expression:
var evenNumbers = numbers.Where(num => num % 2 == 0);
2. Sorting a List of Objects:
Using LINQ:
List<string> fruits = new List<string> { "Apple", "Banana", "Orange", "Grape" };
var sortedFruits = from fruit in fruits
orderby fruit descending
select fruit;
Using Lambda Expression:
var sortedFruits = fruits.OrderByDescending(fruit => fruit);
3. Selecting Specific Properties from a List of Objects:
Using LINQ:
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 25 },
new Person { Name = "Bob", Age = 30 },
new Person { Name = "Charlie", Age = 28 }
};
var names = from person in people
select person.Name;
Using Lambda Expression:
var names = people.Select(person => person.Name);
4. Filtering and Projecting Data from a List of Objects:
Using LINQ:
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 25 },
new Person { Name = "Bob", Age = 30 },
new Person { Name = "Charlie", Age = 28 }
};
var adults = from person in people
where person.Age >= 18
select new { person.Name, person.Age };
Using Lambda Expression:
var adults = people.Where(person => person.Age >= 18)
.Select(person => new { person.Name, person.Age });
5. Grouping Data from a List of Objects:
Using LINQ:
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 25 },
new Person { Name = "Bob", Age = 30 },
new Person { Name = "Charlie", Age = 28 }
};
var ageGroups = from person in people
group person by person.Age < 30 into youngGroup
select new
{
IsYoung = youngGroup.Key,
People = youngGroup.ToList()
};
Using Lambda Expression:
var ageGroups = people.GroupBy(person => person.Age < 30)
.Select(youngGroup => new
{
IsYoung = youngGroup.Key,
People = youngGroup.ToList()
});
These examples demonstrate various scenarios where LINQ and Lambda expressions are used to query, filter, project, sort, and group data in C#. By understanding and mastering these features, developers can write concise and expressive code for data manipulation tasks.
Note: The Person
class used in these examples is assumed to have properties Name
and Age
.
Conclusion:
Both LINQ and Lambda expressions are essential features of C# that offer different approaches to querying and manipulating data. While LINQ provides a declarative and SQL-like syntax for querying data from various sources, Lambda expressions offer a more concise and functional approach for inline delegate functions. Understanding the differences between these two features will help developers choose the appropriate approach based on the specific requirements of their projects
References: