Introduction:
Understanding Cookies and Sessions in Web Development – In web development, managing user data and state is crucial for creating personalized and secure experiences. Two common mechanisms for achieving this are cookies and sessions. In this article, we will explore what cookies and sessions are, their differences, and how they are used in web applications.
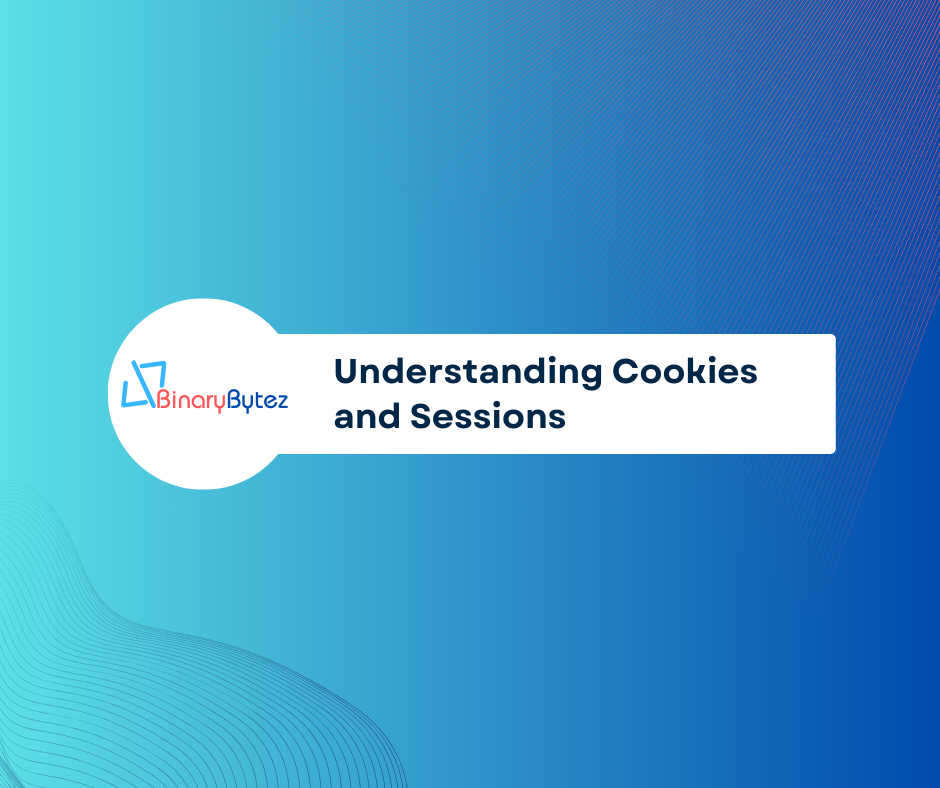
Cookies:
Cookies are small pieces of data stored on the user’s browser. They are sent between the client and the server with each HTTP request, allowing the server to recognize the user. Cookies are typically used for:
- Client-Side Storage: Cookies are stored on the client’s browser, making them an excellent choice for lightweight data that doesn’t require extensive server-side resources.
- Persistent Data: If you need to retain information between sessions and across different pages, cookies are suitable. They have expiration dates that can be set for short or extended periods.
- User Preferences: Cookies are commonly employed for storing user preferences, language settings, or themes, providing a personalized experience.
How Cookies Work:
When a user visits a website, the server sends a Set-Cookie header with the response. The cookie is then stored on the user’s browser. On subsequent requests, the cookie is sent back to the server using the Cookie header.
Set-Cookie: user_id=123; expires=Wed, 01 Jan 2025 00:00:00 GMT; path=/
Here, a cookie named “user_id” is set with an expiration date and a path indicating its scope.
Cookies in ASP.NET Core:
ASP.NET Core provides a convenient way to work with cookies using the HttpContext.Response
and HttpContext.Request
objects.
1. Setting a Cookie:
Cookies are small pieces of data sent by the server and stored on the client’s browser. Here’s how you can set a cookie in an ASP.NET Core controller action:
// In a controller action
public IActionResult SetCookie()
{
// Set a cookie with a key-value pair
Response.Cookies.Append("user_id", "123", new CookieOptions
{
Expires = DateTime.Now.AddDays(7), // Cookie expiration date
HttpOnly = true, // The cookie is not accessible via JavaScript
Secure = true, // The cookie is only sent over HTTPS
SameSite = SameSiteMode.Strict // Restricts the cookie to the same site
});
return View();
}
2. Reading a Cookie:
To read the value of a cookie, use the HttpContext.Request
object in a controller action:
public IActionResult ReadCookie()
{
// Retrieve the value of the "user_id" cookie
string userId = Request.Cookies["user_id"];
// Use the retrieved value as needed
return View();
}
3. Deleting a Cookie:
Deleting a cookie involves setting its expiration date to a past date:
public IActionResult DeleteCookie()
{
// Delete the "user_id" cookie by setting its expiration date to the past
Response.Cookies.Delete("user_id");
return View();
}
Sessions:
Sessions are a way to store user-specific information on the server. Unlike cookies, which are stored on the client-side, sessions exist on the server and are identified by a unique session ID stored in a cookie on the user’s browser. Sessions are typically used for:
- Server-Side Storage: Sessions store data on the server, making them more secure for sensitive information. They are ideal for managing user authentication tokens and other confidential details.
- Temporary Data: If you only need to retain information for the duration of a user’s visit, sessions are well-suited. They automatically expire when the user closes the browser.
- Complex Data Structures: Sessions are preferable when dealing with larger sets of data or complex data structures, as the server handles the storage and retrieval.
How Sessions Work:
- Session Creation: When a user visits a website, a unique session ID is generated, and a session object is created on the server.
- Session ID in Cookie: The session ID is sent to the user’s browser via a cookie.
- Server-Side Storage: The server uses the session ID to retrieve and store user-specific data in the session object.
- Session Expiry: Sessions usually have an expiration time, and when a user remains inactive for a specified period, the session is terminated.
Sessions in ASP.NET Core:
ASP.NET Core provides an abstraction for session management, and it can be configured to use various storage providers, such as in-memory storage, distributed cache, or a database.
1. Enabling Session:
Sessions provide a way to store user-specific information on the server. In the Startup.cs
file, configure services, and add session middleware:
public void ConfigureServices(IServiceCollection services)
{
services.AddDistributedMemoryCache(); // In-memory cache for simplicity, use a more robust solution in production
services.AddSession(options =>
{
options.IdleTimeout = TimeSpan.FromMinutes(30); // Session timeout
options.Cookie.HttpOnly = true; // The session cookie is not accessible via JavaScript
options.Cookie.IsEssential = true; // Mark the session cookie as essential
});
// Other service configurations...
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseSession(); // Enable session middleware
// Other middleware configurations...
}
2. Storing and Retrieving Data in Session:
Now, let’s see how to store and retrieve data in a session:
public IActionResult SetSession()
{
HttpContext.Session.SetString("user_name", "John Doe");
HttpContext.Session.SetInt32("user_age", 25);
return View();
}
public IActionResult ReadSession()
{
string userName = HttpContext.Session.GetString("user_name");
int? userAge = HttpContext.Session.GetInt32("user_age");
// Use the retrieved values as needed
return View();
}
3. Session Timeout:
Adjust the session timeout to control how long session data is retained:
services.AddSession(options =>
{
options.IdleTimeout = TimeSpan.FromMinutes(30); // Session timeout
// ...
});
4. Session Essentiality:
Mark a session cookie as essential to ensure its presence:
services.AddSession(options =>
{
// ...
options.Cookie.IsEssential = true; // Mark the session cookie as essential
});
Differences:
- Location:
- Cookies are stored on the client-side.
- Sessions are stored on the server-side.
- Data Storage:
- Cookies store small amounts of data (4KB per domain).
- Sessions can store larger amounts of data on the server.
- Security:
- Cookies can be manipulated or stolen, posing security risks.
- Sessions are more secure as session data is stored on the server.
Conclusion:
Cookies and sessions are fundamental components of web development, offering ways to manage user data and maintain state. The choice between cookies and sessions depends on the specific requirements of your application. While cookies are suitable for storing small, client-side data, sessions are preferable for managing larger amounts of server-side data. Understanding these mechanisms is essential for creating efficient and secure web applications.