Understanding Clean Architecture is crucial for building maintainable and scalable software. Let’s provide a concise explanation of Clean Architecture:
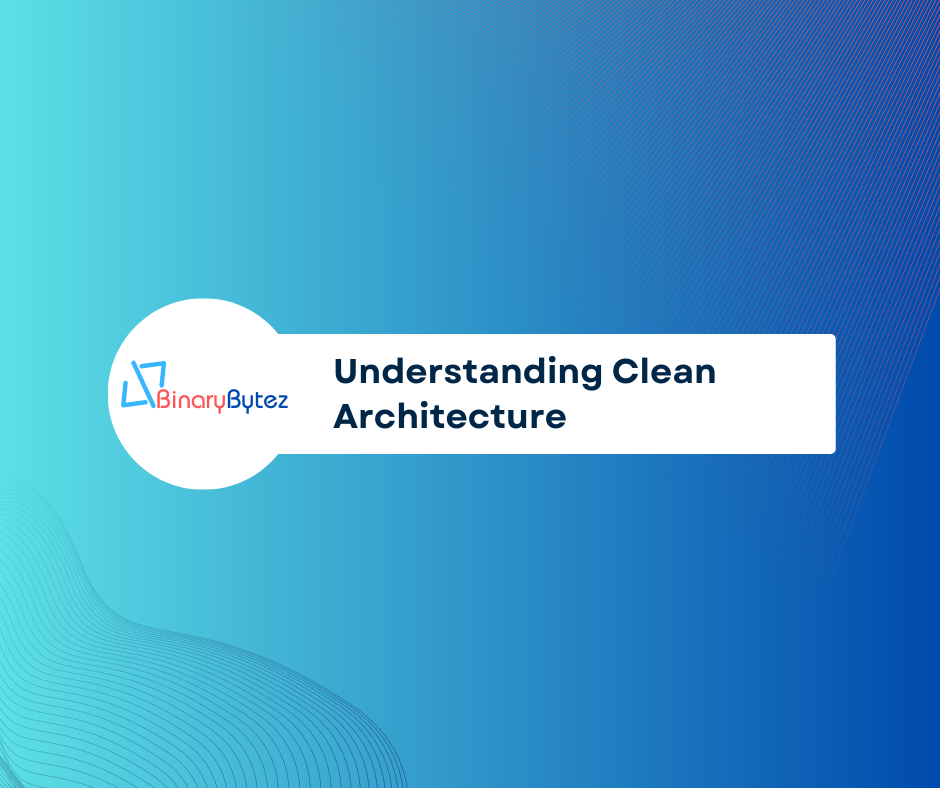
Clean Architecture: A Brief Explanation
Clean Architecture is a software architecture pattern that emphasizes the separation of concerns and the independence of the application’s business logic from external concerns like frameworks, databases, and user interfaces. It was popularized by Robert C. Martin (Uncle Bob) and aims to create a maintainable and flexible codebase that can withstand changes over time.
Key Concepts:
- Separation of Concerns: Clean Architecture promotes dividing the application into distinct layers, each with its specific responsibilities. This separation enables changing one part of the system without affecting the other parts.
- Dependency Rule: The inner layers should not depend on the outer layers. Business logic and core functionality reside in the innermost layer, which should have no knowledge of external frameworks or technologies.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions. This principle fosters a flexible and maintainable codebase by decoupling concrete implementations from abstractions.
- Entities: Core domain models representing business entities exist at the heart of the architecture. They are independent of the external layers and encapsulate core business rules.
- Use Cases (Interactors): Use cases represent application-specific operations or business workflows. They contain the application’s business logic and orchestrate interactions between entities and external layers.
- Interface Adapters: These adapters convert data between the use cases and external components, such as databases, web services, or user interfaces. They allow the use cases to remain independent of external technologies.
- Frameworks and Drivers: The outermost layer is responsible for interacting with external frameworks, databases, and user interfaces. It should not contain business logic but should adapt the data to and from the use cases.
Benefits of Clean Architecture:
- Maintainability: The separation of concerns makes it easier to maintain and modify the application over time, as changes in one part don’t affect the others.
- Testability: Clean Architecture promotes testability by allowing isolated testing of use cases and business rules without involving external dependencies.
- Flexibility: The architecture’s decoupling allows for easy replacement of technologies or frameworks without significant changes to the core application.
- Scalability: The modular structure of Clean Architecture facilitates scaling the application by adding or modifying modules as needed.
- Focus on Business Logic: Clean Architecture helps developers focus on implementing core business rules rather than being bogged down by external concerns.
Sample Structure of the Clean Architecture Project:
├── src
│ ├── Core # Contains the core business logic and domain models, view models, etc.
│ │ ├── Entities # Contains domain models/entities
│ │ │ ├── Product.cs # Sample domain model (can have more entities)
│ │ │ └── ...
│ │ ├── Services # Contains business logic services
│ │ │ ├── IProductService.cs # Sample service interface
│ │ │ ├── ProductService.cs # Sample service implementation
│ │ │ └── ...
│ │ └── ...
│ ├── Infrastructure # Contains infrastructure concerns such as data access, external services, etc.
│ │ ├── Data # Contains data access related classes
│ │ │ ├── ApplicationDbContext.cs # Sample DbContext class
│ │ │ ├── ProductRepository.cs # Sample repository implementation
│ │ │ └── ...
│ │ ├── ExternalServices # Contains code for external services integration
│ │ │ ├── EmailService.cs # Sample external service integration
│ │ │ └── ...
│ │ └── ...
│ └── UI # Contains the user interface layer, including controllers, views, and extensions, etc.
│ ├── Controllers # Contains controllers for handling HTTP requests and responses
│ │ ├── ProductController.cs # Sample controller
│ │ └── ...
│ ├── Views # Contains views for rendering UI components
│ │ ├── Product # Folder for Product-related views
│ │ │ ├── Index.cshtml # Sample view for displaying products
│ │ │ ├── Create.cshtml # Sample view for creating a new product
│ │ │ ├── Edit.cshtml # Sample view for editing an existing product
│ │ │ └── ...
│ │ └── ...
│ └── ...
├── UnitTest
│ ├── Core.Tests # Contains unit tests for the core layer
│ │ ├── ProductServiceTests.cs # Sample unit test for ProductService
│ │ └── ...
│ ├── Infrastructure.Tests # Contains unit tests for the infrastructure layer
│ │ ├── ProductRepositoryTests.cs # Sample unit test for ProductRepository
│ │ └── ...
│ ├── UI.Tests # Contains unit tests for the UI layer
│ │ ├── ProductControllerTests.cs # Sample unit test for ProductController
│ │ └── ...
│ └── ...
└── README.md # Project documentation
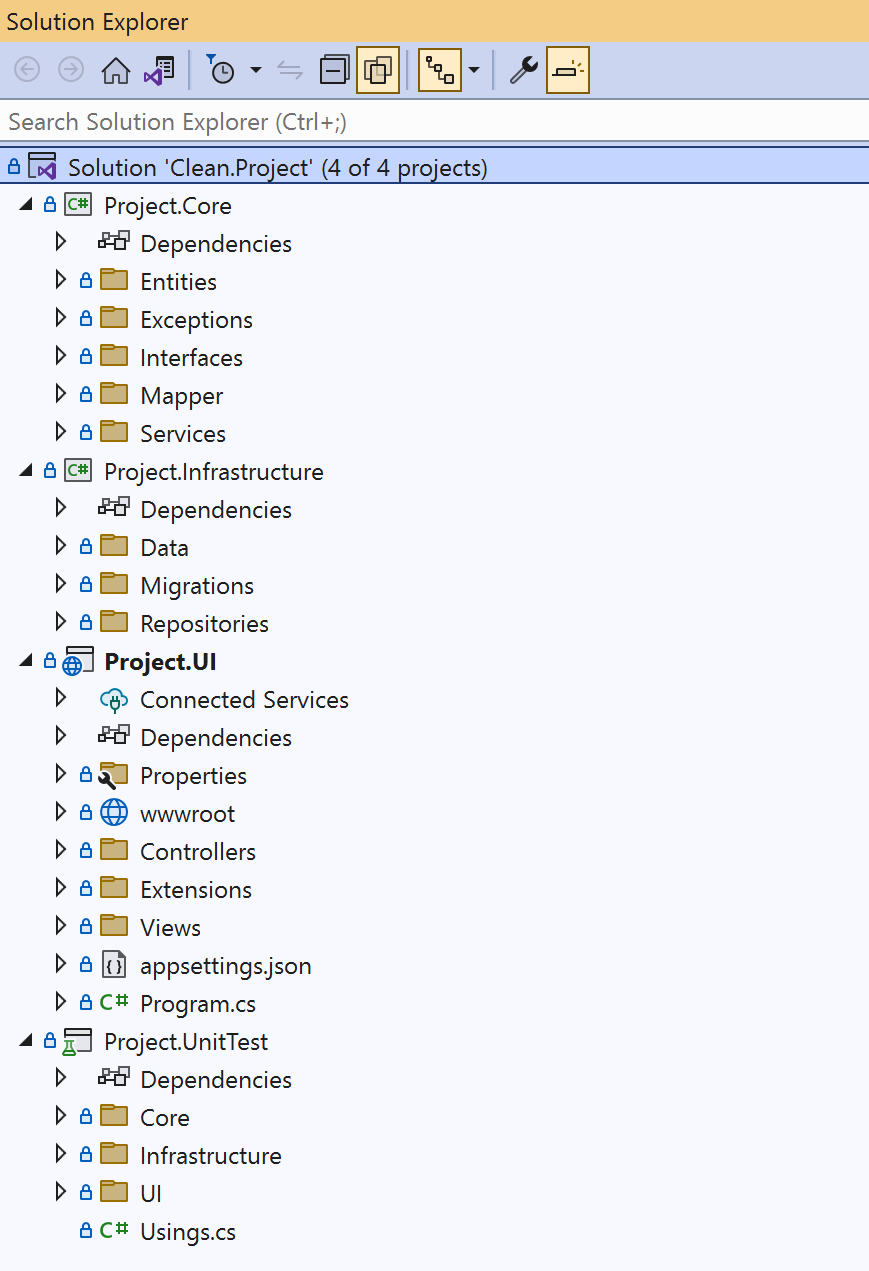
Please note that this is a simplified representation of the project structure, and in a real-world application, you may have more folders and files based on your specific requirements. The above structure adheres to the Clean Architecture principles, with a clear separation of concerns between the core domain logic, infrastructure concerns (data access and external services), and the user interface layer. The tests
folder contains separate test projects for each layer, allowing you to write unit tests to ensure the functionality of each component.
Core:
├── Core
│ ├── Entities # Domain entities representing business objects
│ │ └── User.cs # Example entity class representing a user
│ ├── Services # Business logic and services
│ │ └── UserService.cs # Example service class for user-related operations
│ └── ...
Example Code:
// Core/Entities/User.cs
namespace Core.Entities
{
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
// Other properties and methods relevant to the user entity
}
}
// Core/Services/UserService.cs
using Core.Entities;
using System.Threading.Tasks;
namespace Core.Services
{
public class UserService
{
public async Task<User> GetUserByIdAsync(int userId)
{
// Logic to retrieve user from data source (e.g., database)
}
// Other methods for user-related operations
}
}
Infrastructure:
├── Infrastructure
│ ├── Data # Data access layer
│ │ ├── Repositories # Repository implementations
│ │ │ └── UserRepository.cs # Example repository for user entity
│ │ └── AppDbContext.cs # Entity Framework Core database context
│ └── ...
Example Code:
// Infrastructure/Data/Repositories/UserRepository.cs
using Core.Entities;
using Core.Interfaces;
using Microsoft.EntityFrameworkCore;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace Infrastructure.Data.Repositories
{
public class UserRepository : IRepository<User>
{
private readonly AppDbContext _context;
public UserRepository(AppDbContext context)
{
_context = context;
}
public async Task<IEnumerable<User>> GetAllUsersAsync()
{
return await _context.Users.ToListAsync();
}
// Other CRUD methods for the user entity
}
}
// Infrastructure/Data/AppDbContext.cs
using Core.Entities;
using Microsoft.EntityFrameworkCore;
namespace Infrastructure.Data
{
public class AppDbContext : DbContext
{
public DbSet<User> Users { get; set; }
public AppDbContext(DbContextOptions<AppDbContext> options)
: base(options)
{
}
}
}
API:
├── API
│ ├── Controllers # API controllers
│ │ └── UserController.cs # Example controller for user-related actions
│ ├── Extensions # Extension methods for configuring services
│ └── ...
Example Code:
// API/Controllers/UserController.cs
using Core.Entities;
using Core.Services;
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace API.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class UserController : ControllerBase
{
private readonly UserService _userService;
public UserController(UserService userService)
{
_userService = userService;
}
[HttpGet]
public async Task<ActionResult<IEnumerable<User>>> GetUsers()
{
var users = await _userService.GetAllUsersAsync();
return Ok(users);
}
// Other CRUD actions for user entity
}
}
Unit Testing:
Core.Tests
Infrastructure.Tests
API.Tests
Conclusion:
Clean Architecture is a powerful pattern that promotes code organization, testability, and maintainability. By following its principles, developers can create robust and adaptable software that stands the test of time and can accommodate future changes and enhancements with ease.