Introduction:
In the dynamic world of software development, writing maintainable and scalable code is crucial to the success of any project. SOLID design principles provide a set of guidelines that can help developers achieve precisely that. Developed by Robert C. Martin (Uncle Bob), these principles have become a cornerstone of modern software architecture. In this blog post, we will dive deep into each SOLID principle, understand its significance, and learn how to apply them with practical coding examples.
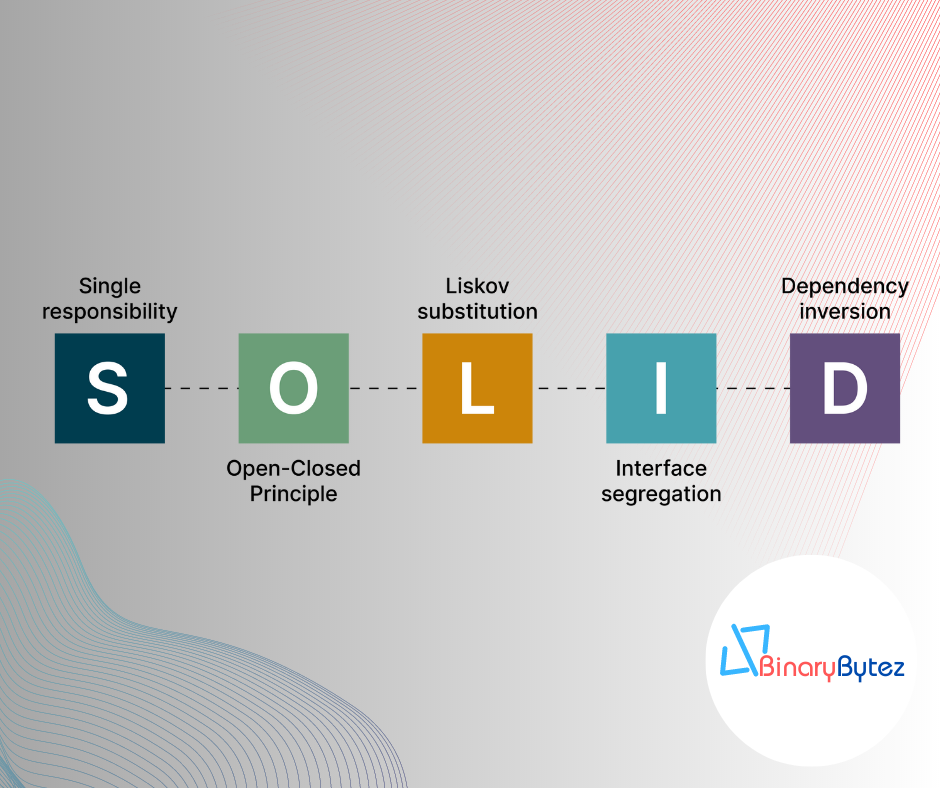
1. Single Responsibility Principle (SRP):
The Single Responsibility Principle states that a class should have only one reason to change. In other words, a class should have a single responsibility or task. Let’s see how we can apply this principle to a sample application.
// Bad Practice - One class with multiple responsibilities
class OrderProcessingService
{
public void ProcessOrder(Order order)
{
// ... Process the order ...
}
public void SendEmailConfirmation(Order order)
{
// ... Send email confirmation ...
}
public void GenerateInvoice(Order order)
{
// ... Generate the invoice ...
}
}
// Good Practice - Separate classes with single responsibility
class OrderProcessor
{
public void ProcessOrder(Order order)
{
// ... Process the order ...
}
}
class EmailService
{
public void SendEmailConfirmation(Order order)
{
// ... Send email confirmation ...
}
}
class InvoiceGenerator
{
public void GenerateInvoice(Order order)
{
// ... Generate the invoice ...
}
}
By following SRP, we ensure that each class has a clear purpose, making the code more modular, maintainable, and easier to understand.
2. Open-Closed Principle (OCP):
The Open-Closed Principle suggests that software entities should be open for extension but closed for modification. This means we should be able to extend the behavior of a class without modifying its existing code. Let’s explore how we can implement this principle.
// Bad Practice - Modifying existing class
class Shape
{
public virtual double Area()
{
// ... Calculate area ...
}
}
class Circle : Shape
{
public override double Area()
{
// ... Calculate circle area ...
}
}
class Square : Shape
{
public override double Area()
{
// ... Calculate square area ...
}
}
// Good Practice - Extending behavior through interfaces
interface IShape
{
double Area();
}
class Circle : IShape
{
public double Area()
{
// ... Calculate circle area ...
}
}
class Square : IShape
{
public double Area()
{
// ... Calculate square area ...
}
}
By using interfaces, we adhere to OCP and create more flexible and adaptable systems that can be easily extended without altering existing code.
3. Liskov Substitution Principle (LSP):
The Liskov Substitution Principle emphasizes that objects of derived classes should be substitutable for objects of the base class without affecting program correctness. Let’s see how we can maintain LSP.
// Bad Practice - Violating LSP
class Bird
{
public virtual void Fly()
{
// ... Fly like a bird ...
}
}
class Penguin : Bird
{
public override void Fly()
{
throw new NotSupportedException("Penguins cannot fly.");
}
}
// Good Practice - Upholding LSP
interface IFlyable
{
void Fly();
}
class Bird : IFlyable
{
public void Fly()
{
// ... Fly like a bird ...
}
}
class Penguin : IFlyable
{
public void Fly()
{
// Penguins cannot fly, but still conform to the interface.
}
}
By following LSP, we ensure that derived classes can seamlessly replace their base class counterparts, promoting code consistency and maintainability.
4. Interface Segregation Principle (ISP):
The Interface Segregation Principle advises segregating interfaces into smaller, focused ones, rather than having large, monolithic interfaces. This promotes a more granular and concise design. Let’s see how to implement ISP.
// Bad Practice - Large, monolithic interface
interface IWorker
{
void Work();
void Eat();
void Sleep();
}
class Robot : IWorker
{
// Implementing unnecessary methods for a robot.
}
class Human : IWorker
{
// Implementing unnecessary methods for a human.
}
// Good Practice - Segregated interfaces
interface IWorkable
{
void Work();
}
interface IEatable
{
void Eat();
}
interface ISleepable
{
void Sleep();
}
class Robot : IWorkable
{
public void Work()
{
// ... Robot work logic ...
}
}
class Human : IWorkable, IEatable, ISleepable
{
public void Work()
{
// ... Human work logic ...
}
public void Eat()
{
// ... Human eat logic ...
}
public void Sleep()
{
// ... Human sleep logic ...
}
}
By adhering to ISP, we create leaner and more focused interfaces, enabling better code maintainability and adaptability.
5. Dependency Inversion Principle (DIP):
The Dependency Inversion Principle suggests relying on abstractions rather than concrete implementations. High-level modules should not depend on low-level modules; both should depend on abstractions. Let’s apply DIP.
// Bad Practice - High-level module depends on low-level module
class OrderProcessor
{
private readonly EmailService _emailService;
public OrderProcessor()
{
_emailService = new EmailService();
}
public void ProcessOrder(Order order)
{
// ... Process the order ...
_emailService.SendEmailConfirmation(order);
}
}
// Good Practice - High-level module depends on abstraction
interface IEmailService
{
void SendEmailConfirmation(Order order);
}
class EmailService : IEmailService
{
public void SendEmailConfirmation(Order order)
{
// ... Send email confirmation ...
}
}
class OrderProcessor
{
private readonly IEmailService _emailService;
public OrderProcessor(IEmailService emailService)
{
_emailService = emailService;
}
public void ProcessOrder(Order order)
{
// ... Process the order ...
_emailService.SendEmailConfirmation(order);
}
}
By following DIP, we promote loose coupling and enable easier testing, extensibility, and a more modular design.
Conclusion:
Incorporating SOLID principles in your software development journey can be transformational. These principles empower developers to write cleaner, more maintainable, and extensible code, which results in robust and scalable software solutions. As you apply SRP, OCP, LSP, ISP, and DIP in your projects, you’ll witness the growth of your coding prowess and the emergence of truly clean code that stands the test of time. Embrace SOLID principles and elevate your coding skills to new heights!