Introduction:
In modern web development, handling concurrency and improving application responsiveness are critical aspects. ASP.NET Core offers powerful tools for managing asynchronous operations through Tasks and Threads. In this blog post, I will delve into the concepts of Tasks and Threads, understand their differences, and explore practical code examples to leverage their benefits in ASP.NET Core applications.
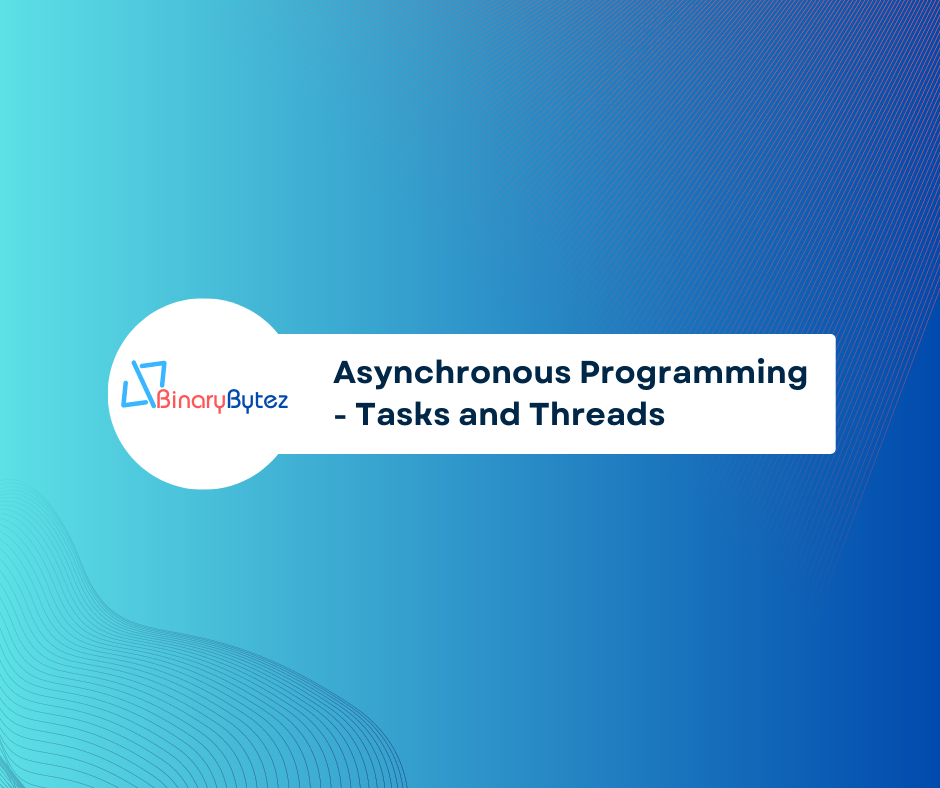
Introduction to Asynchronous Programming:
Asynchronous programming allows applications to perform tasks concurrently, enabling better resource utilization and responsiveness. Unlike synchronous programming, where each operation blocks the thread until completion, asynchronous operations free up threads to handle other tasks. This enhances scalability and improves the overall user experience in web applications.
Understanding Tasks in ASP.NET Core:
Tasks represent asynchronous operations in ASP.NET Core. They encapsulate a unit of work that can run concurrently with other tasks. Here’s an example of creating and running a Task in ASP.NET Core:
using System;
using System.Threading.Tasks;
public class MyService
{
public async Task<string> GetDataAsync()
{
await Task.Delay(2000); // Simulate a time-consuming operation
return "Data from asynchronous operation";
}
}
Exploring Threads in ASP.NET Core:
Threads are low-level constructs used for concurrent programming. While Tasks abstract away the complexities, Threads provide direct control over concurrency. Here’s a basic example of creating and executing a Thread in ASP.NET Core:
using System;
using System.Threading;
public class MyService
{
public void ProcessData()
{
Thread thread = new Thread(DoWork);
thread.Start();
}
private void DoWork()
{
// Perform some work on a separate thread
}
}
Asynchronous Web Requests with Tasks:
In ASP.NET Core, you can use Tasks to perform asynchronous web requests. This ensures that your application remains responsive even during time-consuming API calls. Here’s an example:
using System.Net.Http;
using System.Threading.Tasks;
public class MyService
{
private readonly HttpClient _httpClient;
public MyService(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task<string> GetApiResponseAsync(string url)
{
HttpResponseMessage response = await _httpClient.GetAsync(url);
return await response.Content.ReadAsStringAsync();
}
}
Parallel Processing with Threads:
Threads are suitable for CPU-bound operations that can be executed concurrently. The Parallel
class in ASP.NET Core allows you to execute parallel loops easily:
using System;
using System.Threading.Tasks;
public class MyService
{
public void ProcessDataParallel()
{
Parallel.For(0, 10, i =>
{
// Perform some CPU-bound work in parallel
});
}
}
Best Practices and Considerations:
When working with Tasks and Threads, it’s essential to consider error handling, performance optimization, and choosing the right approach for specific scenarios. Properly managing resources and handling exceptions will ensure a robust and reliable application.
Conclusion:
By understanding and effectively using Tasks and Threads in ASP.NET Core, developers can create responsive, scalable, and high-performance web applications. Whether handling asynchronous web requests or performing parallel processing, mastering these concepts is crucial for building modern web applications.
In conclusion, the power of asynchronous programming in ASP.NET Core lies in the ability to harness the full potential of Tasks and Threads to create efficient and responsive applications. By incorporating these techniques into your development workflow, you can build applications that deliver exceptional user experiences and perform optimally under various conditions.