Introduction:
In the world of modern web development, building robust and efficient APIs is essential for creating scalable and maintainable applications. ASP.NET Core provides a powerful framework for developing Web APIs, and in this blog post, we’ll explore a meticulously crafted ASP.NET Core Web API project template designed to deliver a secure, scalable, and elegant solution.
Whether you’re a seasoned developer or just starting your journey with ASP.NET Core, this project template embodies best coding practices and architectural principles to help you build high-quality APIs with confidence.
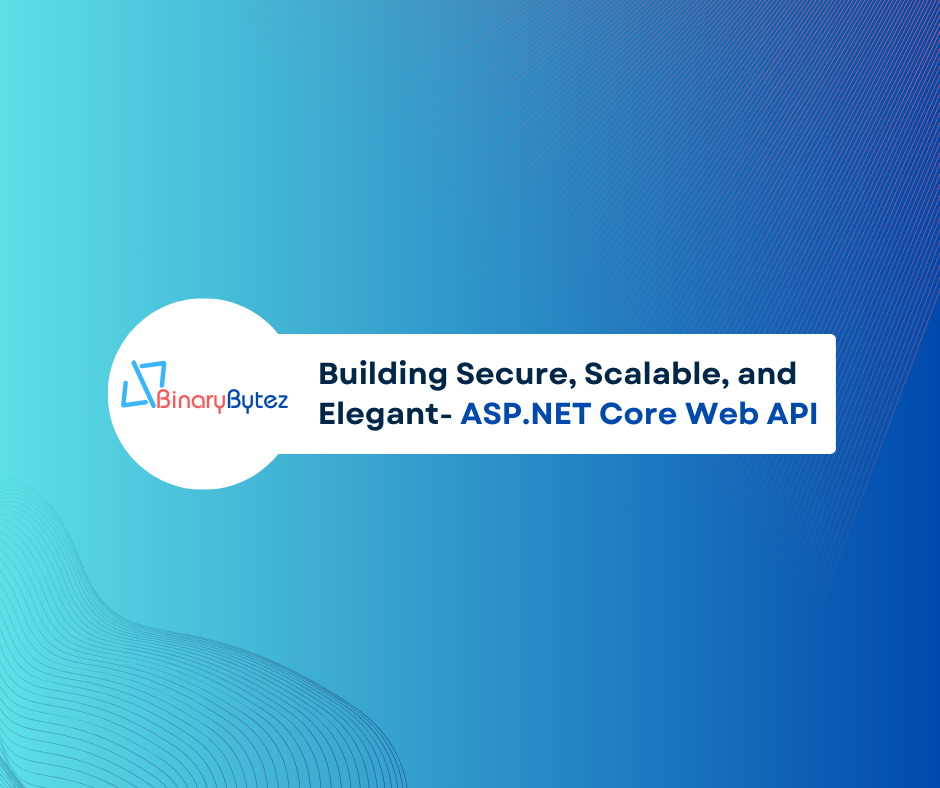
Project Overview:
The ASP.NET Core Web API project template follows a clean architecture pattern, which promotes separation of concerns and modularity. It consists of three main directories:
- Core: Contains the core business logic, including domain models and services.
- Infrastructure: Handles infrastructure concerns such as data access and external services.
- API: Serves as the API layer, including controllers and extensions for handling HTTP requests and responses.
Key Features:
1. Clean Architecture: The project structure is organized to promote a clear separation of concerns, making it easier to understand, test, and maintain the application. Here’s an example of the project structure:
├── src
│ ├── Core
│ ├── Infrastructure
│ └── API
├── tests
│ ├── Core.Tests
│ ├── Infrastructure.Tests
│ └── API.Tests
└── README.md
2. SOLID Design Principles: The codebase follows SOLID principles, enhancing maintainability and extensibility. For example, the UserService
class in the Core layer adheres to the Single Responsibility Principle (SRP) by encapsulating user-related business logic:
// Core/Services/UserService.cs
public class UserService
{
private readonly IUserRepository _userRepository;
public UserService(IUserRepository userRepository)
{
_userRepository = userRepository;
}
public async Task<User> GetUserById(int userId)
{
// Logic to fetch user by Id from repository
return await _userRepository.GetUserById(userId);
}
// Other methods...
}
3. Repository Pattern: The repository pattern abstracts the data access layer and provides a consistent interface for working with data. Here’s an example of a repository class in the Infrastructure layer:
// Infrastructure/Repositories/UserRepository.cs
public class UserRepository : IUserRepository
{
private readonly DbContext _dbContext;
public UserRepository(DbContext dbContext)
{
_dbContext = dbContext;
}
public async Task<User> GetUserById(int userId)
{
// Logic to fetch user by Id from database
return await _dbContext.Users.FirstOrDefaultAsync(u => u.Id == userId);
}
// Other repository methods...
}
4. Entity Framework Core: Entity Framework Core is used as the ORM tool for data access. The ApplicationDbContext
class in the Infrastructure layer represents the database context:
// Infrastructure/Data/ApplicationDbContext.cs
public class ApplicationDbContext : DbContext
{
public DbSet<User> Users { get; set; }
// Other DbSets...
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
// Configure entity mappings...
}
}
5. JWT for Token-based Authentication: Token-based authentication is implemented using JSON Web Tokens (JWT) for managing user sessions, authentication, and authorization securely. Here’s an example of an authentication controller in the API layer:
// API/Controllers/AuthenticationController.cs
public class AuthenticationController : ControllerBase
{
private readonly IAuthService _authService;
public AuthenticationController(IAuthService authService)
{
_authService = authService;
}
[HttpPost("login")]
public async Task<IActionResult> Login(LoginRequestModel model)
{
// Authenticate user and generate JWT token
var token = await _authService.GenerateToken(model.Username, model.Password);
return Ok(new { Token = token });
}
// Other authentication methods...
}
6. API Versioning: API versioning is embraced to support evolutionary changes while maintaining backward compatibility. Here’s an example of versioned controllers in the API layer:
// API/Controllers/V1/UsersController.cs
[ApiController]
[ApiVersion("1.0")]
[Route("api/v{version:apiVersion}/users")]
public class UsersController : ControllerBase
{
// Controller methods for version 1.0 of the API...
}
// API/Controllers/V2/UsersController.cs
[ApiController]
[ApiVersion("2.0")]
[Route("api/v{version:apiVersion}/users")]
public class UsersController : ControllerBase
{
// Controller methods for version 2.0 of the API...
}
7. Dependency Injection: ASP.NET Core’s built-in dependency injection container is used for managing and injecting dependencies throughout the application. Here’s an example of service registration in the Startup class:
// API/Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<IUserService, UserService>();
services.AddScoped<IUserRepository, UserRepository>();
// Other service and repository registrations...
}
8. Unit Testing: Separate test projects are included for unit testing the core, infrastructure, and API layers, ensuring code quality and reliability. Here’s an example of a unit test for the UserService class:
// Core.Tests/Services/UserServiceTests.cs
public class UserServiceTests
{
[Fact]
public async Task GetUserById_ValidId_ReturnsUser()
{
// Arrange
var mockRepository = new Mock<IUserRepository>();
mockRepository.Setup(repo => repo.GetUserById(1)).ReturnsAsync(new User { Id = 1, Username = "testuser" });
var userService = new UserService(mockRepository.Object);
// Act
var result = await userService.GetUserById(1);
// Assert
Assert.NotNull(result);
Assert.Equal("testuser", result.Username);
}
// Other unit tests...
}
Getting Started:
To use this project template, follow these steps:
- Ensure that you have the .NET 7 SDK installed on your machine.
- Clone or download the repository to your local machine.
- Open the solution in your preferred IDE (e.g., Visual Studio, Visual Studio Code).
- Build the solution to restore NuGet packages and compile the code.
- Configure the necessary database connection settings in the appsettings.json file of the Infrastructure project.
- Run the Update-Database command in the Package Manager Console to create the database.
- Run the application by starting the Project.API project.
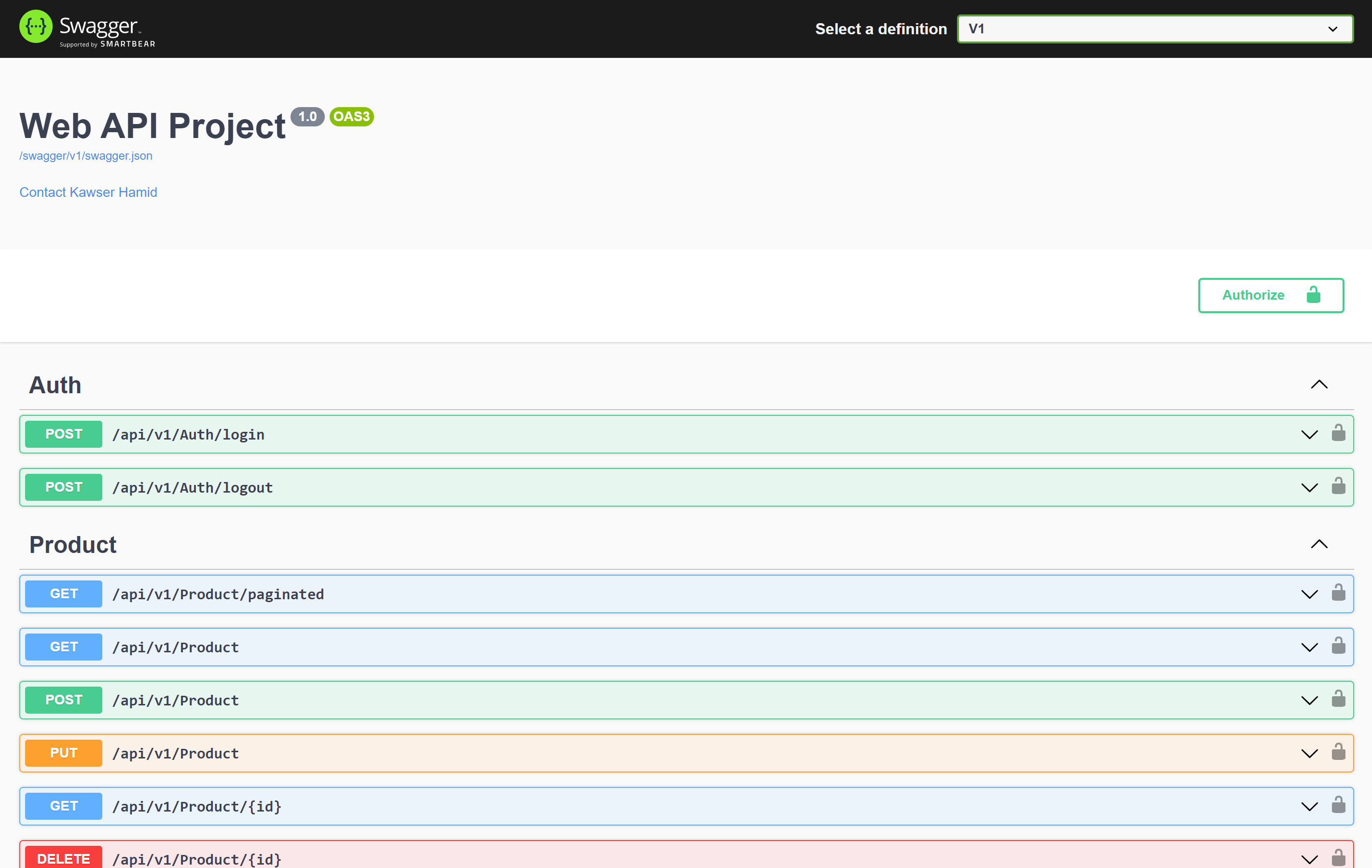
Conclusion:
The ASP.NET Core Web API project template provides a solid foundation for building secure, scalable, and elegant APIs. By following best coding practices, architectural principles, and design patterns, developers can create robust APIs that meet the demands of modern web applications. Whether you’re building a small-scale project or a large enterprise application, this project template equips you with the tools and guidelines necessary for success.