Exception handling is a critical concept in any programming language, ensuring that applications behave predictably even when faced with unexpected issues. In .NET, exception handling helps manage errors or exceptional situations gracefully, allowing developers to handle unforeseen problems and maintain a seamless user experience. In this blog, we’ll explore the details of exception handling in .NET with examples, best practices, and strategies for effective error management.
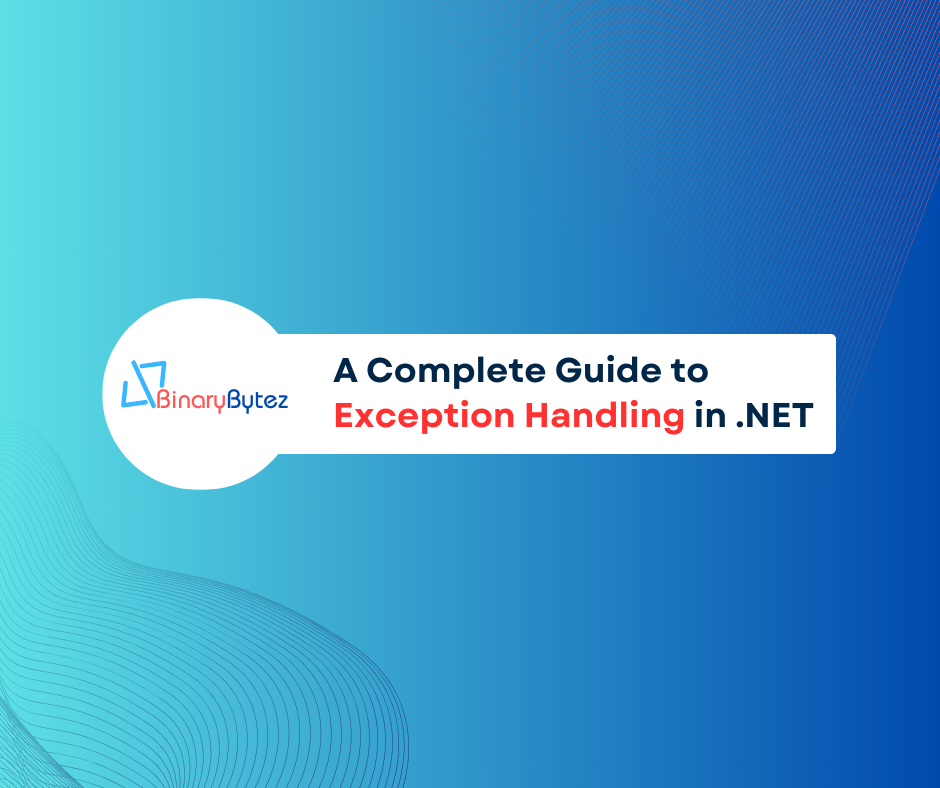
What is an Exception?
An exception is an unexpected event that occurs during the execution of a program, disrupting its normal flow. Exceptions can result from various issues like invalid input, resource unavailability, or runtime errors like dividing by zero.
In .NET, exceptions are objects derived from the System.Exception
class. When an exception occurs, it is thrown (raised), and control is transferred to the nearest exception handler capable of handling it.
Common Types of Exceptions in .NET
Some common built-in exceptions in .NET include:
System.NullReferenceException
: Occurs when an attempt is made to use an object that isnull
.System.IndexOutOfRangeException
: Happens when accessing an index that is outside the bounds of an array.System.DivideByZeroException
: Raised when attempting to divide a number by zero.System.IO.IOException
: Related to Input/Output operations like file handling.System.ArgumentException
: Raised when an argument passed to a method is invalid.
Basic Structure of Exception Handling
In .NET, exception handling is performed using the try
, catch
, finally
, and throw
keywords. Here’s the basic syntax:
try
{
// Code that may throw an exception
}
catch (ExceptionType ex)
{
// Handle the exception
}
finally
{
// Optional block, executes whether an exception occurs or not
}
Example:
public void DivideNumbers(int numerator, int denominator)
{
try
{
int result = numerator / denominator;
Console.WriteLine($"Result: {result}");
}
catch (DivideByZeroException ex)
{
Console.WriteLine("Error: Cannot divide by zero.");
}
finally
{
Console.WriteLine("Operation completed.");
}
}
In this example:
- The
try
block contains the code that may throw an exception. - The
catch
block catches a specific exception (DivideByZeroException
) and handles it. - The
finally
block is optional and runs regardless of whether an exception is thrown.
Throwing Exceptions
You can manually throw exceptions in your code when certain conditions are met. This is done using the throw
keyword.
Example:
public void CheckAge(int age)
{
if (age < 18)
{
throw new ArgumentException("Age must be 18 or above.");
}
Console.WriteLine("Age is valid.");
}
In this case, if the provided age
is less than 18, the method throws an ArgumentException
with a custom message.
Catching Multiple Exceptions
You can handle different types of exceptions by specifying multiple catch
blocks. .NET allows you to catch different exception types and handle them separately.
Example:
public void ReadFile(string filePath)
{
try
{
string content = File.ReadAllText(filePath);
Console.WriteLine(content);
}
catch (FileNotFoundException ex)
{
Console.WriteLine("Error: File not found.");
}
catch (UnauthorizedAccessException ex)
{
Console.WriteLine("Error: Access to the file is denied.");
}
catch (Exception ex)
{
Console.WriteLine("An unexpected error occurred: " + ex.Message);
}
}
In this example:
- The method attempts to read a file.
- It handles specific exceptions like
FileNotFoundException
andUnauthorizedAccessException
. - The final
catch
block catches any other unhandled exceptions.
Using the finally
Block
The finally
block is used to execute code regardless of whether an exception is thrown. It is commonly used to release resources like file handles, database connections, or network connections.
Example:
public void ProcessFile(string filePath)
{
StreamReader reader = null;
try
{
reader = new StreamReader(filePath);
string content = reader.ReadToEnd();
Console.WriteLine(content);
}
catch (IOException ex)
{
Console.WriteLine("An error occurred while reading the file.");
}
finally
{
if (reader != null)
{
reader.Close(); // Ensures the file is closed whether or not an exception occurs
}
Console.WriteLine("File operation completed.");
}
}
In this example:
- The
finally
block ensures that the file is closed whether or not an exception occurs during the file reading process.
Custom Exceptions
You can define your own exceptions by creating custom exception classes that inherit from System.Exception
. This is useful when you need to represent specific error conditions in your application.
Example:
public class InvalidAgeException : Exception
{
public InvalidAgeException(string message) : base(message)
{
}
}
public void ValidateAge(int age)
{
if (age < 0 || age > 120)
{
throw new InvalidAgeException("Age must be between 0 and 120.");
}
Console.WriteLine("Age is valid.");
}
Here, we define a custom InvalidAgeException
and throw it when the age is outside the valid range.
Best Practices for Exception Handling
- Use Specific Exceptions:
- Always catch the most specific exception that applies to your situation (e.g.,
FileNotFoundException
instead ofException
).
- Don’t Swallow Exceptions:
- Avoid empty
catch
blocks that suppress exceptions without logging or handling them. Always log or handle exceptions in a meaningful way.
catch (Exception ex)
{
// Bad practice: Swallowing the exception
}
- Use
finally
for Cleanup:
- If your
try
block allocates resources (like file handles or database connections), ensure those resources are properly released using thefinally
block.
- Avoid Using Exceptions for Flow Control:
- Exceptions should be used to handle exceptional situations, not as a mechanism for controlling regular program flow.
// Avoid doing this:
try
{
// Code that might throw an exception for expected scenarios
}
catch
{
// Handle expected scenarios with exceptions
}
- Provide Useful Error Messages:
- When throwing or logging exceptions, provide meaningful messages to help identify and debug the problem.
- Handle Exceptions at the Right Level:
- Catch exceptions at the appropriate level in your application. For example, handle user input errors in the UI layer and database connection errors in the data access layer.
- Rethrow Exceptions When Necessary:
- Sometimes you need to catch an exception, perform some action, and then rethrow it to be handled elsewhere.
catch (Exception ex)
{
// Perform some action
throw; // Rethrow the exception
}
Global Exception Handling
In some cases, especially in larger applications, it’s useful to implement global exception handling. In a .NET application, this can be done in the Main
method (for console apps) or by using middleware (for ASP.NET Core apps).
Global Exception Handling in Console Apps
public static void Main(string[] args)
{
try
{
// Application code
}
catch (Exception ex)
{
Console.WriteLine("An unhandled exception occurred: " + ex.Message);
}
}
Global Exception Handling in ASP.NET Core
In ASP.NET Core, you can create middleware to handle exceptions globally.
public class ExceptionHandlingMiddleware
{
private readonly RequestDelegate _next;
public ExceptionHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (Exception ex)
{
// Handle the exception and return a user-friendly error response
context.Response.StatusCode = 500;
await context.Response.WriteAsync("An internal server error occurred.");
}
}
}
Conclusion
Exception handling is an essential aspect of writing robust and reliable .NET applications. By using structured exception handling, following best practices, and implementing custom exceptions where necessary, you can ensure that your applications are more resilient, maintainable, and user-friendly. Proper error management not only helps prevent crashes but also provides meaningful feedback to both developers and users, improving the overall quality of your software.
Remember to catch specific exceptions, avoid swallowing errors, and implement global exception handling where necessary. With these strategies in place, you’ll be well-prepared to handle the unexpected in your .NET applications.