In the world of programming, understanding interfaces and abstract classes in C# is essential for creating flexible and maintainable code. In this blog post, we’ll explore these foundational concepts, discussing their definitions, differences, benefits, and providing clear examples in C#. Let’s dive into the basics of interfaces and abstract classes in C# programming!
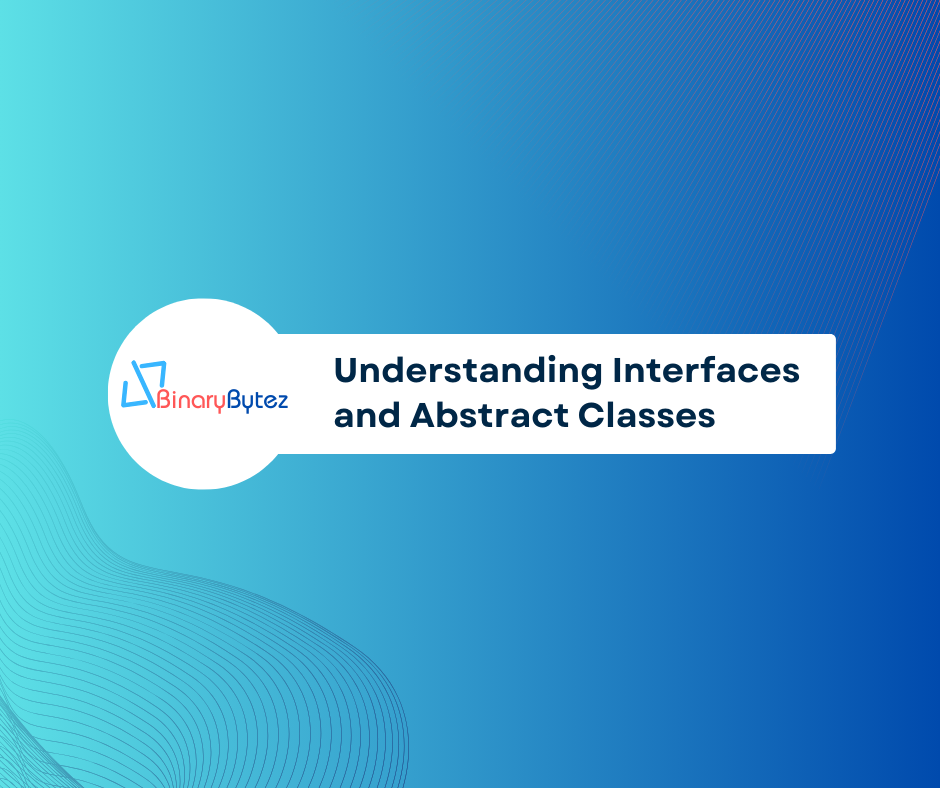
Interfaces:
Definition:
Interfaces in C# serve as contracts that define a set of methods or properties that implementing classes must adhere to. They declare the “what” of a class without specifying the “how,” promoting loose coupling and allowing for polymorphism. Interfaces are declared using the interface
keyword and can be implemented by multiple classes.
Syntax:
Interfaces are declared using the interface
keyword.
interface IAnimal {
void MakeSound();
}
Example:
Let’s consider a scenario where we have different animals in a zoo. We want each animal class to implement a method to make a sound. We can define an interface IAnimal
:
interface IAnimal {
void MakeSound();
}
Each animal class can then implement this interface and provide its own implementation of the MakeSound
method:
class Dog : IAnimal {
public void MakeSound() {
Console.WriteLine("Woof");
}
}
class Cat : IAnimal {
public void MakeSound() {
Console.WriteLine("Meow");
}
}
Benefits:
Abstract Classes:
Definition:
Abstract classes in C# are classes that cannot be instantiated on their own and may contain abstract methods (methods without implementation) as well as concrete methods (methods with implementation). They provide a blueprint for derived classes to follow, enabling code reuse and providing a common base for related classes.
Syntax:
Abstract classes are declared using the abstract
keyword.
abstract class Shape {
public abstract double GetArea();
}
Example:
Let’s consider a drawing application where we have different shapes, each with its own area calculation logic. We can define an abstract class Shape
:
abstract class Shape {
public abstract double GetArea();
}
Each shape class can inherit from this abstract class and provide its own implementation of the GetArea
method:
class Rectangle : Shape {
public double Length { get; set; }
public double Width { get; set; }
public override double GetArea() {
return Length * Width;
}
}
class Circle : Shape {
public double Radius { get; set; }
public override double GetArea() {
return Math.PI * Radius * Radius;
}
}
Benefits:
Differences and Considerations:
Here’s a tabular format highlighting the differences between interfaces and abstract classes in C#:
Feature | Interfaces | Abstract Classes |
---|---|---|
Instantiation | Cannot be instantiated directly | Cannot be instantiated directly |
Inheritance | Supports multiple inheritance | Does not support multiple inheritance |
Members | Can only contain method signatures (no fields or implementation) | Can contain both abstract and concrete members (including fields and methods with implementation) |
Implementation | All members are abstract (no implementation) | Can have both abstract and concrete members |
Purpose | Defines a contract for classes to implement | Provides a blueprint for derived classes |
Example Syntax | csharp interface IExample { void Method(); } | csharp abstract class Example { public abstract void Method(); } |
This tabular format clearly outlines the key differences between interfaces and abstract classes in C#, making it easier to understand their distinctions.
In conclusion, interfaces and abstract classes are powerful tools in C# for designing flexible and maintainable code. Understanding their differences and benefits is crucial for effective software design and development.